Scout Copilot
A step-by-step guide to creating and launching Scout Copilot on your website
Introduction to Scout Copilot
The Copilot brings the powerful functionality of Scout directly to your website or application, providing an interactive assistant that enhances user experience and engagement. This guide will take you through every step of creating and launching a Scout Copilot, from setting up workflows and customizing the Copilot’s appearance to embedding it seamlessly into your web environment. Whether you’re introducing your audience to AI-driven insights or offering an interactive support tool, this guide provides everything you need to make Scout Copilot a valuable part of your digital experience.
New to Scout? Watch our quick-start demo to get familiar with the platform:
Using Copilot Blocks in a Workflow
Each Scout Copilot is powered by a workflow which has special blocks that are specifically designed to interact with the Copilot client. Generally, this is the minimum basic workflow template you can start with:
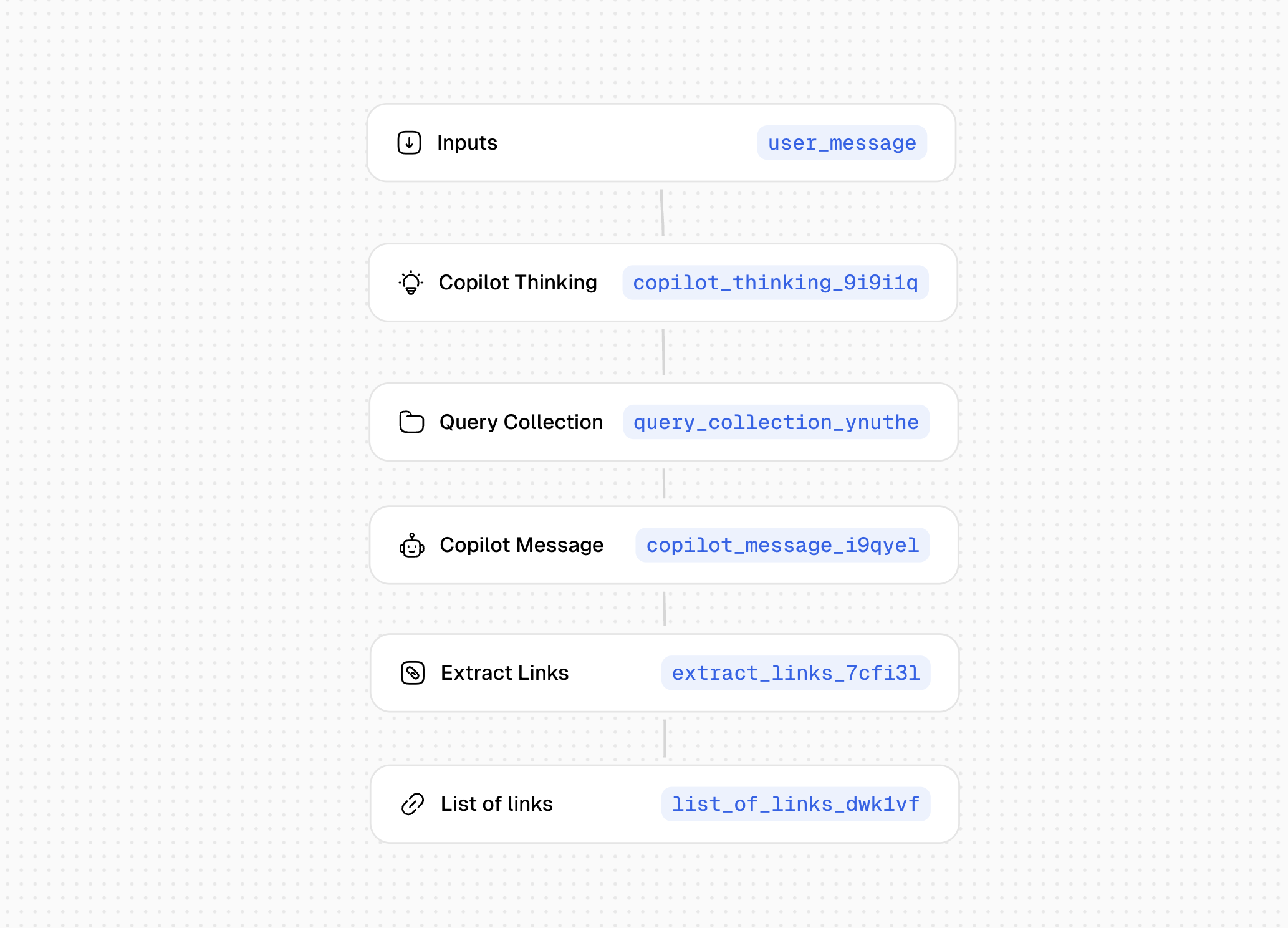
We will continue to invest and expand into these blocks, and other programmatic ways to interact with the Copilot. However, today these are the key blocks interactions to understand:
Input Block
The Copilot sends the user’s chat message to the inputs.user_message
input by default.
Copilot Thinking Block
This sends a “pending state” message to the Copilot client, giving the user context on the action being taken during that step of execution in the workflow that is generating the Scout Copilot response.
Copilot Message Block
This is similar to an LLM block. The connection to the Scout Copilot in the client is streaming, so you can send many messages in one workflow run using subsequent Copilot message blocks.
If you are using the list of links block mentioned below, consider adding the following to your system
prompt:
Query Collection Block, Extract Links Block, and List of Links Block
The Query Collection Block can be used in a normal fashion. However, you can pair it with the Extract Links and List of Links blocks to include beautified citations at the end of the Copilot message block output.
To use these together, you can pass your COPILOT_MESSAGE_BLOCK_ID.output
directly to the Extract Links block, which will extract and beautify any links contained in the collection query response.
Then, you pass the EXTRACT_LINK_BLOCK_ID.output
to the List of Links block, and it will append the formatted list of sources to the Copilot in the client.
Creating a Copilot
You can create a Copilot from the integrations tab by clicking ”+ New Copilot.”
Once you click into a created Copilot in the Scout dashboard, you can select the workflow that powers the Copilot and customize images, colors, sizes, and text content of the Copilot.
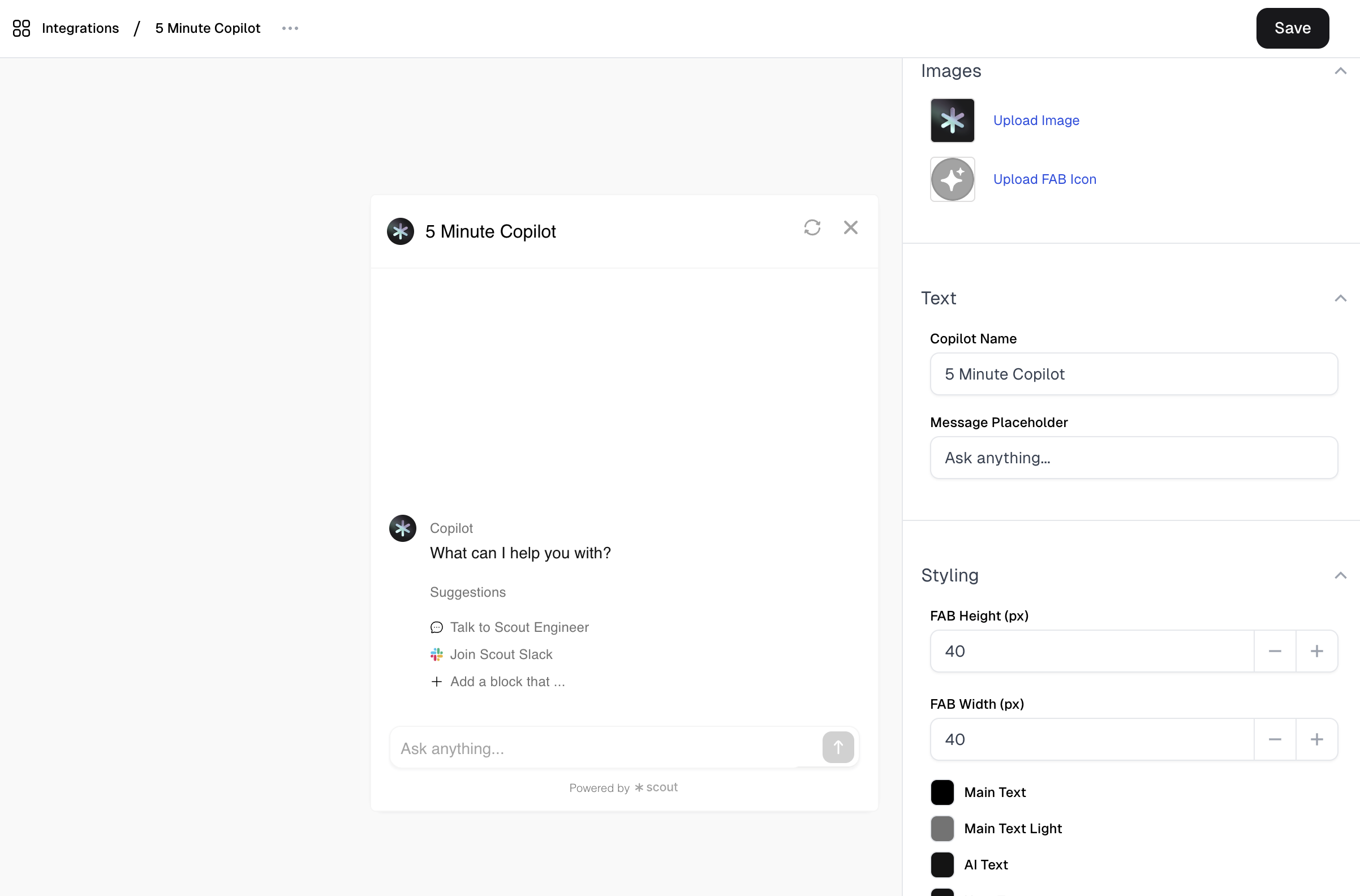
Getting a 401? Make sure you set the Settings > Allowed Origins
appropriately in the Copilot settings within the Scout dashboard. For example, if you’re testing from localhost:8000
, you will want to add http://localhost:8000
to your allowed origins. Similarly, do this for any website domains where you will be installing the Copilot.
Adding the Copilot Widget to Your Website
To incorporate the Copilot module into your application, insert the following code:
After these steps, the Copilot component will be visible on your website.
Below, you’ll find descriptions of different attributes that can be applied to the Copilot component. These attributes can be added directly to the scout-copilot
tag.
For instance, to conceal the Copilot, you can set the show
attribute to false
:
Required Attributes
The copilot_id
is the unique identifier of the Copilot, available on the Copilot’s detail page. This ID is required for proper functionality.
Optional Attributes
Specifies the Copilot’s environment, which defaults to "development"
. Set this to "production"
when deploying your application.
Identifies the user interacting with the Copilot. If not set, a random ID will be generated and stored in local storage.
Determines whether the component is visible. The default value is true
.
Indicates whether the Copilot is open. It defaults to false
.
Specifies whether the minimize button is visible, with a default value of true
.
The height of the Copilot component. The default value is "40vh"
.
The width of the Copilot component. The default value is "25vw"
.
Specifies the maximum width of the message bubble. Defaults to "95%"
.
The height of the floating action button (FAB). Defaults to "40px"
.
The width of the FAB. Defaults to "40px"
.
Determines whether the Copilot is embedded directly into the application interface, rather than appearing as a floating component. It defaults to false
. See the Embedding the Copilot section for more information.
Customizing the FAB
The Copilot’s floating action button (FAB) can be customized by passing in a child HTML element with the slot
attribute set to fab
. For example:
In the example above, we are creating a simple FAB with a white background, a shadow, and an image and text inside. You can customize the FAB to your liking by changing the styles and content.
Embedding the Copilot
By default, the Copilot will be displayed as a floating action button (FAB) in the bottom right corner of the screen. To embed the Copilot within your website, set the embedded
attribute to true
. This will allow you to position the Copilot within your website’s layout wherever you see fit.
Note: When embedding the Copilot, the open
, show
, and show_minimize
attributes will be overridden.
In the example above, the Copilot is embedded within the .container
div and it is given a width of 700px and a height of 500px.
Using Initial Activities
The initial_activities
property allows you to set up the initial state of the Copilot chat by preloading messages and actions when the Copilot is initialized.
This feature is useful for customizing the user’s first interaction with the Copilot, such as displaying a welcome message or suggesting common queries.
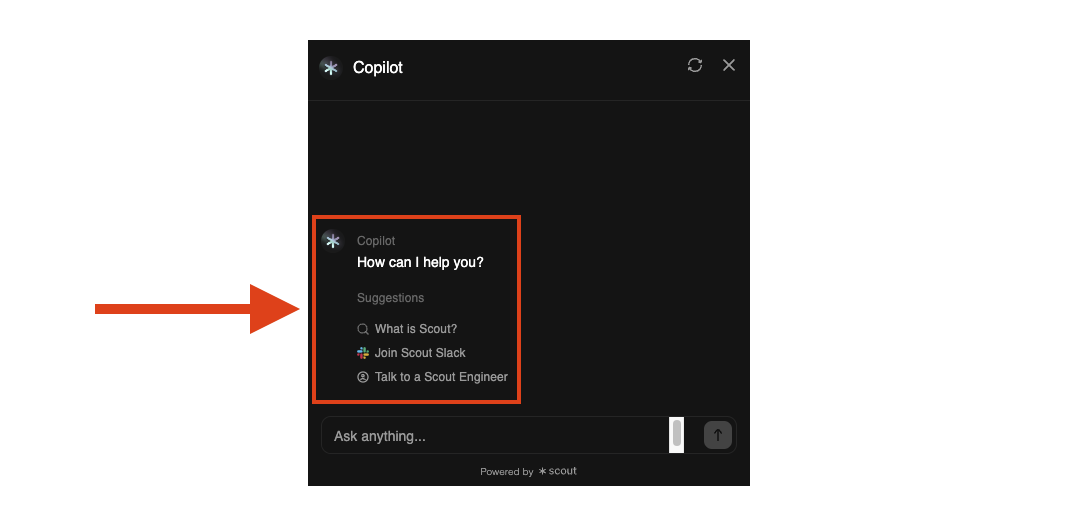
You can pass initial_activities
to the Copilot component via JavaScript after it has loaded. The initial_activities
is an array of activity objects that define messages or actions to be displayed in the chat interface.
Here is an example of how to set initial_activities
:
In this example, the Copilot will display an assistant message saying “How can I assist you today?” followed by a list of suggested actions, such as common queries or links to resources.
Activity Types
The initial_activities
array can include the following activity types:
-
llm.chat.message
: Represents a chat message from the assistant or user.role
: The role of the message sender, either'assistant'
or'user'
.content
: The text content of the message.img_url
: (Optional) URL of the avatar image for the message sender.
-
action_list
: Displays a list of actionable items to the user.header
: The header text for the action list.items
: An array of action items.
Action Item Types
Within an action_list
, the items
array can include the following action item types:
-
suggested_query
: A suggested question or command that the user can click to send to the assistant.title
: The display text for the suggestion.query
: The query text that will be sent to the assistant when clicked.img_url
: (Optional) URL of an icon to display alongside the suggestion.
-
link
: A link to an external resource.title
: The display text for the link.url
: The URL to navigate to when clicked.img_url
: (Optional) URL of an icon to display alongside the link.
By customizing initial_activities
, you can tailor the initial user experience of the Copilot to better suit your application’s needs.
Implementing the Copilot in React
If you are building your application using React and Next.js, you can integrate the Copilot component seamlessly. Below is an example of how to implement the Copilot in a React application using TypeScript.
In this example:
- We include
'use client';
at the top to indicate that this is a client-side component in Next.js. - We import
Script
from'next/script'
to load the external Copilot script. - We render the
<scout-copilot>
element within the component. - We use the
onLoad
prop of theScript
component to set theinitial_activities
after the script has loaded. - We cast the
scout-copilot
element to include theinitial_activities
property.
To use this component in your application, simply import and include it in your JSX:
Note: Ensure that your project is configured to support custom HTML elements in JSX. You may need to adjust your TypeScript configuration or include custom type definitions.